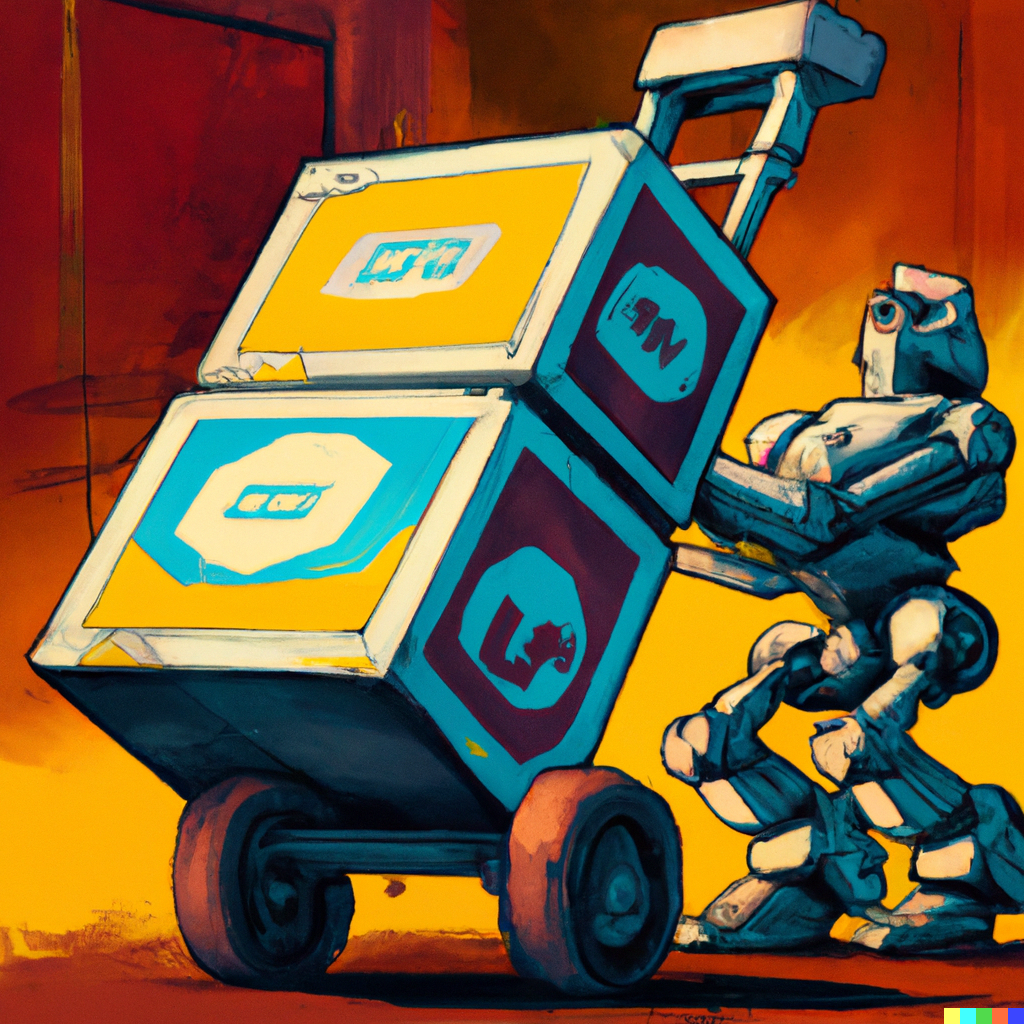
In this post we are going to develop an IVR load test using TestIVR
In the previous article I discussed how you can implement an IVR experience test using TestIVR API. I also discussed how you can implement an IVR feature test using TestIVR API. In this post I am going to discuss IVR Load Testing and how you can implement an IVR Load Test using TestIVR.
IVR load testing, evaluates the IVR system performance under large number of simultaneous calls. Basically large number of test calls should be made to the IVR system to evaluate its performance at expected traffic volumes. A good load test starts with lower number of simultaneous calls and gradually increase the number to the expected traffic volume. By running load tests on regular basis you can make sure the available resources (bandwith, CPU, memory, etc.) is enough to handle the expected volume of calls and your IVR system can provide an acceptable experience for your customers at all time. So let's discuss how we can implement an IVR load test using TestIVR.
After signing up the TestIVR web portal will provide you with an "API key" which can be used to call the TestIVR RESTful API. Using the API key you can call the TestIVR load testing tool via different tools such as "curl", python libraries such as "requests".
The TestIVR API end point is https://api.testivr.com/
.
Keep in mind that in all your API calls to TestIVR API endpoint, you should add your "API key" in a Header called "Authorization" and you need to add "Api-Key
" at its begining.
So for instance in "curl" you will have something like (replace XXXXX
with your "API key"):
curl -X POST \ -H "Content-Type: application/json" \ -H "Authorization: Api-Key XXXXX" \ -d '{ "description":"sample path" }' https://api.testivr.com/path/
Or in python:
import requests headers = { 'Content-Type': 'application/json', 'Authorization': 'Api-Key XXXXX', } json_data = { 'description': 'sample path', } response = requests.post('https://api.testivr.com/path/', headers=headers, json=json_data)
Similar to experience test, to develop an IVR load test using TestIVR,
you should start with creating a "path".
As discussed in the previous article, a "path" is basically a route for your test to follow.
Later you can add "action"s (such as pressing a button or saying something to the IVR) to this path.
Here I am going to use the same path and actions I created in the previous article.
The id
of that path was "3".
So we can take a look at that path by submitting a GET
request to path/
endpoint and passing 3/
as the url parameter:
curl --request GET \ -k \ --url https://api.testivr.com/path/3/ \ --header 'Authorization: Api-Key XXXXX'
The API will response with something like this:
{ "count":1, "next":null, "previous":null, "results":[ { "id":3, "created_at":"2022-10-24T23:15:41.262451Z", "description":"path to sales", "user":1 } ] }
To see the "action"s created on path "3" you can submit a GET
request to action/
endpoint and
pass the path id as a parameter like ?path=3
:
curl --request GET \ -k \ --url https://api.testivr.com/action/?path=3 \ -H "Authorization: Api-Key XXXXX"
And the response will be:
{ "count":4, "next":null, "previous":null, "results":[ { "id":3, "created_at":"2022-10-24T23:17:34.962940Z", "order":0, "type":"listen", "value":"Hello, how can we direct your call? Press 1 for sales, or say sales. To reach support, press 2 or say support.", "description":"listening to the ivr intro", "asr_threshold":0.9, "pause_before":0.0, "pause_after":0.0, "user":1, "path":3 }, { "id":4, "created_at":"2022-10-24T23:22:34.685174Z", "order":1, "type":"press", "value":"1", "description":"press 1", "asr_threshold":0.9, "pause_before":0.0, "pause_after":1.0, "user":1, "path":3 }, { "id":5, "created_at":"2022-10-24T23:24:28.167247Z", "order":2, "type":"listen", "value":"You asked for sales, please hold", "description":"listening to the prompt", "asr_threshold":0.9, "pause_before":0.0, "pause_after":1.0, "user":1, "path":3 },{ "id":7, "created_at":"2022-10-25T00:45:29.329226Z", "order":3, "type":"command", "value":"terminate", "description":"terminating the call", "asr_threshold":0.9, "pause_before":0.0, "pause_after":0.0, "user":1, "path":3 } ] }
In the above actions, the test basically first listens for an intro from the IVR system. Then it presses number 1 on the keypad. Then it listens for another prompt, and at the end it terminates the call. You can read more detail about how we created the above actions in the previous article.
The next step is to setup the load test using the defined path.
You can do that by submitting a POST
request to /load/test/
endpoint.
Important parameters to pass are:
path
: the path you want to use for your test.phone
: the phone number of the IVR you want to run the test oncalls
: the total number of calls you want to run for the testlevels
: the total number of levels you want to run for the test
TestIVR will run several levels of calls through the the provided phone
number.
The number of levels you want to run should be passed via the levels
parameter.
In each level, TestIVR will make multiple simultaneous calls, and the number of calls will increase in each level.
The total number of calls in all levels will be equal to the value passed as calls
parameter above.
TestIVR will calculate the number of calls in each level based on the number of levels
and the number of calls
.
For instance if you pass "600" for calls
and "3" for levels
, TestIVR will make 3 levels of calls to the IVR system.
In the first level it will make 100 simultaneous calls to the IVR system, after all those 100 calls are completed it will move to the next level.
In the second level TestIVR will make 200 simultaneous calls and after those are completed, in the final level it will make the remaining 300 calls.
So in our case I am going with:
curl -X POST \ -k \ --url https://api.testivr.com/load/test/ \ --header "Content-Type: application/json" \ --header "Authorization: Api-Key XXXXX" \ --data '{ "name": "sales path load test ", "description":"load test for sales path", "path": 3, "phone": "16506839455", "levels": 3, "calls": 6 }'
And the result will be like this:
{ "status": "OK", "data": { "id": 1, "created_at": "2022-11-10T00:35:28.796403Z", "description": "load test for sales path", "name": "sales path load test", "phone": "16506839455", "levels": 3, "calls": 6, "user": 1, "path": 3 } }
From above you need to keep in mind what is the id
for your new load test, which in this case is 1.
Now the load test will run, it may take a while since we have multiple levels of calls to go through. Keep in mind that each run will have separate set of outcomes, a run can fail due to various reason such as IVR system declining the call, or the promopt doesn't match the expected values.
If you need to pull the outcomes you need to first pull the test
id, to do that you can submit a GET
request to /load/run/
endpoint:
curl -X GET \ -k \ --url https://api.testivr.com/load/run/ \ --header "Content-Type: application/json" \ --header "Authorization: Api-Key XXXXX"
The output goning to be like this:
{ "count":6, "next":null, "previous":null, "results":[ { "id":6, "created_at":"2022-11-10T00:36:50.789522Z", "user":1, "load_test":1, "test":15 },{ "id":5, "created_at":"2022-11-10T00:36:50.523642Z", "user":1, "load_test":1, "test":14 }, ... { "id":1, "created_at":"2022-11-10T00:35:29.053003Z", "user":1, "load_test":1, "test":10 } ] }
You see for the load_test
with id "1" we have total of six results, each for one of the calls made to the system.
To see the outcome
of each test you need its test
id.
So for instance to see the outcome
of the test
with id "15" (first one above), you can submit the following request:
curl -X GET \ -k \ --url https://api.testivr.com/outcome/?test=15 \ --header "Content-Type: application/json" \ --header "Authorization: Api-Key XXXXX"
Note that we are filtering the outcomes based on the test id by adding ?test=15
to the request.
The result will be like:
{ "count":2, "next":null, "previous":null, "results":[ { "id":16, "created_at":"2022-11-10T00:37:05.484793Z", "outcome":"match", "value":"hello how can we direct your call press one for sales or say sales to reach support press two or say support", "expected_value":"Hello, how can we direct your call? Press 1 for sales, or say sales. To reach support, press 2 or say support.", "match_ratio":0.908256880733945, "user":1, "test":15, "action":3 }, { "id":19, "created_at":"2022-11-10T00:37:15.510006Z", "outcome":"match", "value":"you asked for sales please hold", "expected_value":"You asked for sales, please hold", "match_ratio":0.9523809523809523, "user":1, "test":15, "action":5 } ] }
In the response, each items of the resuls
shows the outcome of one of the "actions" we had on the "path".
We can see the "action" id in the action
field of the response.
This article shows how you can create a simple load test for IVR using TestIVR. Please let me know if you have any question through our email: support@testivr.com
We also provide a good document on our API which provides more detailed information on all the calls you can make to TestIVR.